Basic Python commands
Learn Python easily. Discover simple Python commands for beginners. This guide covers important functions and language rules in Python for beginners.
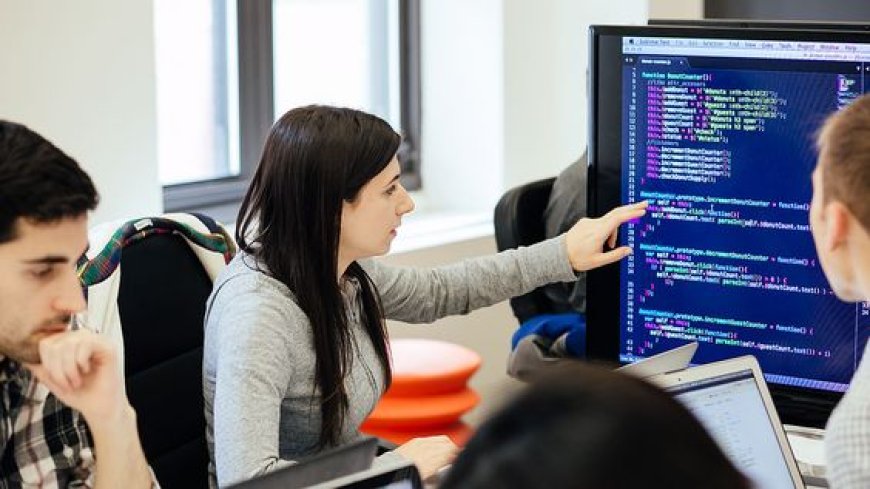
Learning Python can be both exciting and a bit challenging, especially if you're new to programming. Python is a widely used language for various tasks, like making websites and working with data. However, to become good at it, you need to understand the basics. This guide is here to help beginners get a solid grasp of the essential Python commands, setting the foundation for further learning.
In this Python tutorial, we'll cover the basics of Python programming, including simple things like how to write code and work with different types of information. Knowing these basics is important if you want to get good at Python. We'll also talk about Python libraries and frameworks, which are tools that make it easier to do more advanced things with Python. By the end of this guide, you'll have a good understanding of how Python works, and you'll be ready to learn more advanced stuff.
Understanding the Print Statement in Python
Python is known for being easy to read and understand, and one of the things it makes simple is printing stuff on the screen using the 'print' statement. Whether you're just starting with Python or you've been coding for a while, knowing how to use the print statement well is important for writing good code. Let's go over the basics and also some fancier stuff you can do with it.
# Basic Print Statement
print("Hello, Python!")
# Printing Variables
name = "John"
age = 25
print("Name:", name, "Age:", age)
# Formatting Output
amount = 100.456
print("Amount: {:.2f}".format(amount))
# Concatenating Strings
first_name = "John"
last_name = "Doe"
print("Full Name:", first_name + " " + last_name)
Besides the basics, Python also gives you some cool ways to make your printing look nice. For example, you can use f-strings:
# Using f-strings
name = "Alice"
age = 30
print(f"Name: {name}, Age: {age}")
You can also change how things are separated or ended in your printouts using 'sep' and 'end':
# Customizing output
print("One", "Two", "Three", sep=", ", end="!")
Whether you're fixing problems in your code or making things easier for people who use your programs, being good at using the print statement in Python is important. As you get better at it, you might want to check out some other tools like PyCharm, Jupyter Notebooks, or VS Code to help you code even better.
Variables and Data Types in Python
Python programming language is known for its flexibility and robust features, making it a favorite among data scientists and programmers. Let's dive into the basics of variables and data types in Python, understanding their importance in both general programming and data science.
In Python, variables act like containers to store data values. Unlike some other languages, Python doesn't need you to declare variables upfront. You simply assign a value, and Python figures out the data type automatically. This dynamic typing makes coding more straightforward and flexible.
Python supports different data types:
1. Numeric Types: These include Integers (`int`) and floating-point numbers (`float`) for dealing with numerical data.
For example:
x = 10
y = 3.14
2. Strings: Strings (`str`) are used for sequences of characters and are enclosed in single or double quotes. They're handy for handling textual data:
name = "John Doe"
3. Lists: Lists (`list`) are ordered collections of items, which can be of different data types. They're changeable, making modifications easy:
numbers = [1, 2, 3, 4, 5]
4. Tuples: Tuples (`tuple`) are like lists but unchangeable; once defined, their elements can't be altered:
coordinates = (10, 20)
5. Dictionaries: Dictionaries (`dict`) store key-value pairs, allowing efficient data retrieval based on keys:
person = {"name": "Alice", "age": 30, "city": "New York"}
Understanding these basic data types and variables sets a strong foundation for Python programming, especially in data science. Python's versatility in handling different data types makes it a powerful tool for both data scientists and programmers alike.
Basic Arithmetic Operations in Python: A Foundation for Advanced Concepts
Python programming, knowing how to do simple math is important. It's like laying the foundation for doing more complicated stuff later on. Python is known for being easy to use and can do a lot of different things. There are some really helpful libraries in Python, like NumPy, SciPy, and Pandas. These make it easy for people who work with data and machine learning to do calculations without much trouble. Even in web development, Python is used in frameworks like Django and Flask to do math for websites.
Learning basic math in Python is not just useful for programming, though. It's also super helpful for people who want to work with data or do machine learning. Exploring the different Python tools and libraries in Python can help you do more advanced things and solve problems better. Whether you're into analyzing data, making machine learning programs, or building websites, knowing how to do basic math in Python is important. It's like the first step in getting good at using Python, and it opens up a lot of possibilities for what you can do next.
Mastering Conditional Statements in Python: A Comprehensive Guide
Conditional statements are fundamental to programming, and Python offers powerful tools to implement them efficiently. Here’s a breakdown of key points to master them:
-
Syntax: Understand the syntax of conditional statements in Python, including if, Elif, and else statements.
-
Boolean Logic: Familiarize yourself with Boolean expressions to evaluate conditions effectively.
-
Indentation: Remember that Python relies on indentation to define blocks of code within conditional statements.
-
Comparison Operators: Learn how to use comparison operators such as ==, !=, <, >, <=, and >= to compare values.
-
Logical Operators: Explore logical operators like and, or, and not to combine multiple conditions.
-
Nested Conditionals: Understand how to nest conditional statements within each other for more complex decision-making.
-
Ternary Operator: Discover the concise ternary operator syntax for simple conditional expressions.
Understanding Function Definitions in Python
Function definitions in Python are like magic tools for making code bits you can use over and over. They help bundle instructions into named chunks, making your code easier to understand and reuse. Let's break down what you need to know about function definitions:
1. How to Write Them: In Python, you start a function with the word `def`, then give it a name and put any needed info in parentheses. The code that belongs to the function is indented below.
2. Using Them Again and Again: Once you make a function, you can call it as many times as you want from anywhere in your code. This saves you from writing the same instructions over and over.
3. Giving Them Info: Functions can take in info, which we call parameters. These let you run the same code but with different values. Parameters can be basic, like numbers, or more specific, like names.
4. Getting Stuff Back: Functions can also give back info using the `return` word. This lets them hand off results to other parts of your code.
The Input Function in Python for Better User Interaction
Python is a great tool for making interactive apps, and one important feature is the input function. Let's take a closer look at how it works and why it's essential for user interaction in Python.
1. Easy Syntax: The input function in Python is easy to use. You just write `input(prompt)`. The `prompt` is like a friendly message asking the user to type something.
2. Getting User Input: When you use the input function, your program takes a break and waits for the user to type something on the keyboard. After they press Enter, the input becomes a string that the program can use.
3. Handles Different Inputs: The input function is smart! It can deal with different types of input, like numbers or words, depending on what the user types.
4. Useful Everywhere: Whether you're making a simple text-based program or a cool game, the input function is a basic tool for talking with users in all kinds of Python projects.
Dealing with Mistakes in Python: Using Try-Except Blocks
Fixing mistakes in your code is important when you're programming. In Python, there's a cool tool called a try-except block that helps you smoothly do this. In any programming language, mistakes happen, but how you handle them can make a big difference in how well your code works. The try-except block is like a safety net for your code. It lets you catch any mistakes that might happen while your code is running and deal with them without crashing your whole program.
Here's how it works:
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError:
# Handling the specific exception
print("Error: Division by zero")
In this example, if Python tries to divide by zero (which it can't do), instead of crashing, it jumps to the code inside the except block and prints an error message.
You can also handle different kinds of errors in different ways:
try:
# Code that may raise an exception
result = int(input("Enter a number: "))
except ValueError:
# Handling specific exception
print("Error: Invalid input. Please enter a valid number.")
except Exception as e:
# Handling any other unforeseen exception
print("An unexpected error occurred:", e)
By using try-except blocks like this, you can make your Python code more reliable and user-friendly. Learning how to handle mistakes gracefully is an important skill for any Python developer.
Exploring Basic File Operations in Python
Working with files is a basic but crucial part of programming, especially when dealing with data. Python comes with handy tools and libraries that make file operations simple and flexible. Whether you want to read from or write to files, Python has straightforward methods to get the job done.
Reading from a file:
# Open a file in read mode
with open('example.txt', 'r') as file:
# Read the entire contents of the file
data = file.read()
print(data)
Writing to a file:
# Open a file in write mode
with open('example.txt', 'w') as file:
# Write data to the file
file.write("Hello, world!")
Appending to a file:
# Open a file in append mode
with open('example.txt', 'a') as file:
# Append data to the file
file.write("\nAppending new content!")
Using the `with` statement ensures that files are closed properly, preventing memory issues.
Python supports additional file operations, like reading line by line (`readline()`), reading all lines into a list (`readlines()`), and more advanced tasks like seeking within a file. Understanding these operations is vital for any developer, as it allows efficient handling of data stored in files a common need in programming. With Python's user-friendly syntax and robust library support, mastering file operations becomes simple and enjoyable.
In summary, learning the basics of function definitions in Python is essential for becoming proficient in programming. When you grasp how to create functions, deal with inputs and outputs, use docstrings for explanations, and handle variable scope, you can design code that is both modular and reusable. Python's focus on simplicity and readability makes it a great language for both beginners and experienced programmers. With these fundamental commands in your toolkit, you'll be ready to tackle a variety of programming tasks and explore the possibilities of Python development.