How to Write Python Code for Beginners
Discover how to write Python code if you're just starting. Follow along with easy, step-by-step tutorials to learn the basics. Get started coding in Python today
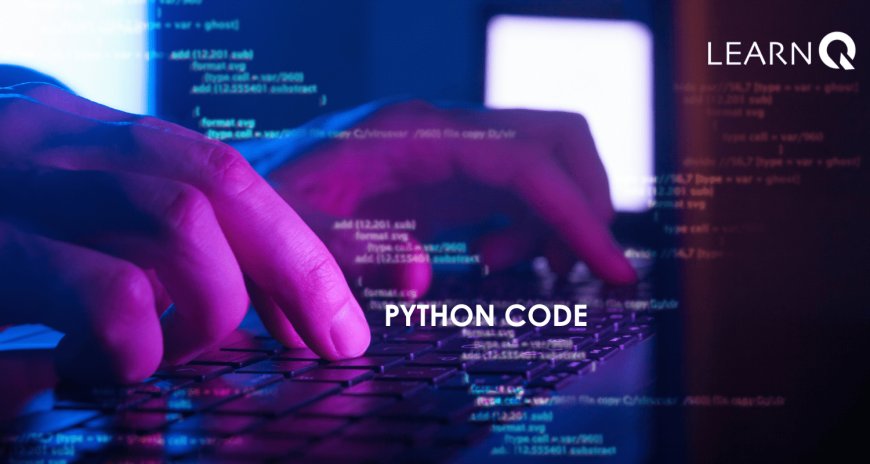
Making decisions based on data and the exciting advancements in artificial intelligence, Python stands out as a fantastic programming language. It's easy to use, versatile, and comes with lots of tools, making it great for different areas like data science, data analytics, and AI. Whether you're just starting or a pro wanting to boost your skills, mastering Python can be a game-changer.
This guide is here to help you learn Python coding with a focus on what you need for data science, data analytics, and artificial intelligence. From the basics of Python to advanced techniques for handling data and using machine learning, we've got it covered. Whether you want to analyze big sets of data, create models that predict things, or build smart systems, knowing Python is crucial. we'll use practical examples, step-by-step Python tutorials, and best practices to make sure you can write code that's not only powerful but also easy to understand. By the end, you'll feel confident and ready to tackle real-world challenges in data science, analytics, and AI using Python. Get ready to explore Python with us and discover how it can open up a world of possibilities for innovation and problem-solving. Let's dive into the exciting journey of mastering Python for data and AI.
Exploring Python Programming: Unveiling its Importance and Learning Path
Python is a widely used programming language known for its simplicity and usefulness. Many people, including developers, data scientists, and educators, prefer Python because it's easy to read and has a large community of users. As the demand for Python skills grows, it's essential to grasp advanced concepts to stay competitive in the software development field. What makes Python special is not just its simplicity but also its ability to handle complex tasks gracefully. Whether you're into web development, machine learning, or other areas, knowing Python opens up various opportunities. As businesses increasingly rely on data-driven insights, the demand for Python programming skills becomes more significant.
To meet this demand, there are comprehensive Python courses available. These courses cover a range of topics, starting from basic syntax to advanced libraries and frameworks. You might learn about object-oriented programming, data structures, algorithms, web development with Django or Flask, scientific computing with NumPy and SciPy, and machine learning with TensorFlow or PyTorch. Additionally, online resources and interactive Python platforms make learning hands-on and accessible. This allows anyone, regardless of their background, to explore Python programming at their own pace.
Example Python Code:
# Example: Calculate the factorial of a number
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
number = 5
print("Factorial of", number, "is", factorial(number))
This Python code example demonstrates how to calculate the factorial of a number, showcasing the practical side of what you can achieve with Python programming.
Understanding the Challenges: Problems You Might Face When Learning Python Programming
Technology, Python is popular. It's used by lots of developers for things like working with data, making machines learn, and building websites. But learning Python and all the stuff that goes with it isn't easy. Let's look at some of the problems you might run into:
1. Lots of Different Python Tools: Python has a bunch of different tools that developers use. But figuring out which ones you need can be hard, especially when you're just starting. You have to spend time figuring out which tools are best for things like working with data or building websites.
2. Harder Python Stuff: Python is known for being easy to read and write. But when you get into the more advanced stuff like decorators or generators, it gets tough. Learning these things is important for writing good code, but it takes time and practice.
3. Different Ways to Build Websites: If you want to build websites with Python, there are a bunch of different ways you can do it. Each way has its own rules and things to learn. Picking the right way and understanding how it works can be tricky.
4. Getting Different Tools to Work Together: Sometimes, when you're working on a project, you need to use different Python tools together. But making them all work together smoothly can be hard. You might run into problems like some tools not working well together or needing different versions of the same tool.
5. Learning About Data and Machines: Python is popular for working with data and making machines learn. But to do that, you need to understand things like statistics and algorithms. This can be tough, especially if you're new to it.
How Can I Write Python Code Effectively?
To write Python code effectively, start by making it easy to read and understand. Follow the PEP 8 style guide, which gives rules for how to format your code neatly. Break your code into smaller parts, like functions or classes, to make it easier to work with and reuse. Use clear names for your variables and functions so that others (and future you) can understand what they do. Use tools like Git to keep track of changes and work with others on your code. Use programs like PyCharm or VSCode to help you write your code faster and find mistakes. Write comments in your code to explain what it does, so anyone reading it can understand. And keep learning new things about Python so you can keep getting better at writing code that works well. Following these steps will help you write Python code that's clean, efficient, and easy to work with.
Guide to Becoming Proficient in Python
Python is a useful programming language used in lots of different areas like making websites, working with data, and making smart machines. Getting good at Python takes some practice and a plan. Here's a simple roadmap to help you learn Python step by step:
1. Basics First
Begin by learning the basic stuff in Python, like how to write code, use different kinds of information, and control what your program does. Here's a simple example:
# Example Python code for basic concepts
# Variables and Data Types
x = 10
name = "John"
is_student = True
# Control Structures
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
# Functions
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
2. Data Structures
Get to know the different ways Python can store information, like lists, dictionaries, and more. Here's a quick look:
# Example Python code for data structures
# Lists
numbers = [1, 2, 3, 4, 5]
print(numbers[0]) # Accessing elements
# Dictionaries
person = {"name": "Alice", "age": 30, "city": "New York"}
print(person["name"]) # Accessing values
3. Object-Oriented Programming (OOP):
Learn about objects and how Python can use them to make things happen in your programs. Here's a simple example:
# Example Python code for OOP
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print("Hello, my name is", self.name)
person = Person("Bob", 25)
person.greet()
4. File Handling:
Learn how to make Python read and write information in files on your computer. Here's how you can do it:
# Example Python code for file handling
# Writing to a file
with open("example.txt", "w") as file:
file.write("Hello, World!")
# Reading from a file
with open("example.txt", "r") as file:
data = file.read()
print(data)
5. Modules and Packages:
Find out how Python can use other people's code to help you in your programs. Here's how you can do it:
# Example Python code for using modules and packages
# Creating a module
# mymodule.py
def greet(name):
print("Hello, " + name + "!")
# Using the module
import mymodule
mymodule. greet("Alice")
6. Error Handling:
Learn how to help your Python programs deal with problems without crashing. Here's how:
# Example Python code for error handling
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero!")
7. Advanced Topics:
Once you're comfortable, explore more advanced topics like working with lots of things at once, searching for patterns in text, and more. Here's a simple example:
# Example Python code for advanced topics
# Using Regular Expressions
import re
text = "Hello, my email is example@email.com"
match = re.search(r'[\w\.-]+@[\w\.-]+', text)
if match:
print("Email found:", match.group())
8. Projects and Practice:
The best way to get good at Python is to practice a lot! Try making your projects and solving fun coding challenges. Keep at it, and you'll get better and better!
Following these steps and practicing regularly will help you become good at Python and open up lots of exciting possibilities for you in the world of coding. Have fun learning!
In conclusion, getting good at writing Python code is a journey that involves learning and practicing regularly. By understanding the basics, following good practices, and exploring advanced concepts, you can improve your coding skills. Python is great for both beginners and experienced developers because it's easy to read and versatile. Remember, coding isn't just about making programs that work it's about creating solutions that are efficient, easy to maintain, and elegant. Stay curious, connect with the active Python community, and always look for chances to make your skills better. With dedication and a love for getting better, you can make the most of Python to handle different programming challenges.